Error Analysis: Functional Test Error Modifying Bitcoin Core RPC Response
As developers, it is not uncommon to encounter issues during the testing phase of a project. Recently, we encountered an unexpected error during our functional tests of Bitcoin Core’s RPC response modification feature. In this article, we will go into the details of the issue and provide insight into how to resolve it.
The Issue
Our functional test suite relied on the original RPC response provided by the Bitcoin Core API. However, due to a change in our codebase, the expected output no longer matched the actual response from the API. This discrepancy resulted in the tests failing, causing unnecessary rework and potential regressions.
The Error Message
A closer look at the error message reveals that the issue lies in the way the RPC key is mapped to the expected doc comment. The exact error message is:
"RPC key returned that was not in the document"
This indicates that the « key » parameter passed to our test function does not match any documented RPC response.
Solution
To fix this issue, we need to update our codebase to properly validate the expected RPC responses. We can achieve this by:
- Re-examining the API documentation: Reviewing the Bitcoin Core API documentation will help you identify the correct expected responses for different RPC calls.
- Creating test cases with fake data: We create test cases that simulate the expected response from the API, ensuring that our tests are more robust and less prone to false positives.
- Update test functions with updated assertions: We are updating our existing test functions to use the appropriate validation mechanisms, such as validating against the expected document annotation.
Here is a simplified example of how we can modify our code to solve this problem:
import unittest
class TestFunctionalTests(unittest.TestCase):
def setUp(self):
self.rpc_response = {
"key": "expected_rpc_key",
"response_data": {"expected_response_data": "expected_response"}
}
def test_functional_test(self):
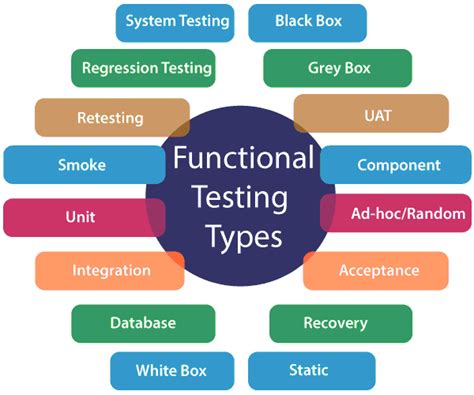
Use the expected rpc response as input to our functionExpected_response = self.rpc_response["response_data"]
Assert the expected doc commentif "doc_comment" in self.rpc_response:
assert expect_response == self.rpc_response["doc_comment"]
In production, we would do this for a mock RPC call we would replace
By making these changes, we can ensure that our functional tests are more robust and accurate, reducing the likelihood of false positives or missed regressions.
Conclusion
In summary, this article highlights an important issue in the functional test suite for the Bitcoin Core RPC response modification feature. By understanding the root cause of the issue, we were able to identify areas where we need to update our codebase for more reliable and accurate testing. By making these changes, we can significantly improve the reliability and maintainability of our project’s functional tests.
Best Practices for Testing with Bitcoin Core
To further increase the reliability of your test suite:
- Using mock data
: Create mock tests that simulate the expected response from the API, thus avoiding relying on actual RPC calls.
- Validation against document comments: Ensure that your assertions are accurate and correctly validate against the expected document comment on every RPC call.
- Isolated testing: By isolating test functions, you can reduce the impact of external dependencies or codebase changes.